Building a custom dynamic pie chart component
How to build a dynamic component, working with data model properties
After reading this article, you’ll know:
- How to build dynamic components
- How to add data model properties to your component
Pre-requisites
To create and update your content, you need to understand the syntax and logic of the following coding languages and libraries:
- Javascript (ES6)
- React
We recommend using Visual Studio Code as your code editor to create custom components and steps as this is what we use in our guides.
We will work further on the already created pie chart in the previous article. If you haven’t followed this article already, check out the tutorial on how to make this pie chart component here.
Adding input fields
To make our pie chart dynamic, we need to be able to add data model properties as values. Since the prefab is the part where the user can add values, we will change that part.
To add data model properties we will need type: VARIABLE:
{
type: 'VARIABLE',
label: 'Amount 1',
key: 'amount',
value: ['1'],
},
It allows the user to select properties from the data model. This variable also needs a title, for that we can use the type: ‘TEXT’:
{
type: 'TEXT',
label: 'Title 1',
key: 'title',
value: 'Type your title here...'
},
After we’ve added this to our prefab, it now looks like this:
(() => ({
name: "Hello World",
icon: "TitleIcon",
category: "CONTENT",
structure: [
{
name: "text",
options: [
{
type: 'TEXT',
label: 'Title 1',
key: 'title',
value: 'Type your title here...'
},
{
type: 'VARIABLE',
label: 'Amount 1',
key: 'amount',
value: ['1'],
},
{
type: 'COLOR',
label: 'Color 1',
key: 'color',
value: 'Primary'
},
{
type: 'TEXT',
label: 'Title 2',
key: 'title1',
value: 'Type your title here...'
},
{
type: 'VARIABLE',
label: 'Amount 2',
key: 'amount1',
value: ['1'],
},
{
type: 'COLOR',
label: 'Color 2',
key: 'color1',
value: 'Primary'
},
],
descendants: []
}
]
}))();
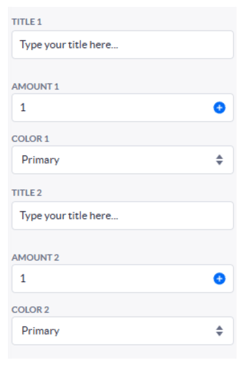
We can now change the “amount” to a data model property using the blue button (+), while still being able to fill in our own static values.
Adding properties to the pie chart
The next thing we need to do is to convert the properties to readable numbers, to do this we will take the text from key: amount 1+2
using const { useText } = B;
and useText()
, with the right value selected, we can convert it into a number using Number
.
Add in JSX underneath the recharts:
jsx: (
<div className={classes.root}>
{(() => {
const {
Recharts: {
PieChart,
Pie,
Cell,
ResponsiveContainer,
}
} = dependencies;
const { useText } = B;
const amount = Number(useText(options.amount));
const amount1 = Number(useText(options.amount1));
We will now add a check if the numbers given are greater than 0, otherwise, the chart will just disappear.
const hasValidData = amount > 0 || amount1 > 0;
In the following code, we’ll set the value to the corresponding title. When one of the given values is greater than 0, the given amount will be set; otherwise, the default value of 1 will be returned.
This is because when adding a data model property, the value given isn’t a number yet when you are in the builder, only after compiling, the chart will get access to the property value.
const data01 = hasValidData
? [
{ name: options.title, value: amount },
{ name: options.title1, value: amount1 }
]
: [
{ name: options.title, value: 1 },
{ name: options.title1, value: 1 }
];
To show our data we can use a label in our <pie>
. The label adds a line with the given value next to the chart showing the needed information. Let's add a label to our code and give it the right property.
<ResponsiveContainer width="100%" height={300}>
<PieChart width={730} height={250}>
<Pie
data={data01}
cx="50%"
cy="50%"
outerRadius={80}
label={({ index }) => data01[index].name}
>
<Cell className={classes.root} />
<Cell className={classes.root1} />
</Pie>
<Tooltip/>
</PieChart>
</ResponsiveContainer>
In this code, we have also added <Tooltip/>, <Tooltip/> can show more information about the values in the chart, in our case the name and amount. We only need to import it from Recharts:
imports: [
'PieChart',
'Pie',
'Cell',
'ResponsiveContainer',
'Tooltip'
],
And add it to our constant:
const {
Recharts: {
PieChart,
Pie,
Cell,
ResponsiveContainer,
Tooltip
}
} = dependencies;
Now our code should look like this:(() => ({
name: 'text',
type: 'BODY_COMPONENT',
allowedTypes: [],
orientation: 'HORIZONTAL',
dependencies: [
{
label: 'Recharts',
package: 'npm:recharts@2.15.0',
imports: [
'PieChart',
'Pie',
'Cell',
'ResponsiveContainer',
'Tooltip'
],
},
],
jsx: (
<div className={classes.root}>
{(() => {
const {
Recharts: {
PieChart,
Pie,
Cell,
ResponsiveContainer,
Tooltip
}
} = dependencies;
const { useText } = B;
const amount = Number(useText(options.amount));
const amount1 = Number(useText(options.amount1));
const hasValidData = amount > 0 || amount1 > 0;
const data01 = hasValidData
? [
{ name: options.title, value: amount },
{ name: options.title1, value: amount1 }
]
: [
{ name: options.title, value: 1 },
{ name: options.title1, value: 1 }
];
return (
<ResponsiveContainer width="100%" height={300}>
<PieChart width={730} height={250}>
<Pie
data={data01}
cx="50%"
cy="50%"
outerRadius={80}
label={({ index }) => data01[index].name}
>
<Cell className={classes.root} />
<Cell className={classes.root1} />
</Pie>
<Tooltip/>
</PieChart>
</ResponsiveContainer>
);
})()}
</div>
),
styles: B => theme => {
const style = new B.Styling(theme);
return {
root: {
fill: ({ options: { color } }) => style.getColor(color),
},
root1: {
fill: ({ options: { color1 } }) => style.getColor(color1),
},
};
},
}))();
Our pie chart is ready for testing, back in the page builder we can add our new chart and see our changes:
As expected, we don’t see our property values, but when we compile the page, we’ll see that the values “2” and “6” are added to the chart. We can check by hovering our mouse over the chart; our tooltip will show the title and value.